Dive into Investment Research - A Beginner’s Guide to the OpenBB Platform
The OpenBB Platform allows you to access financial data from various providers. We often use it for our investment research.
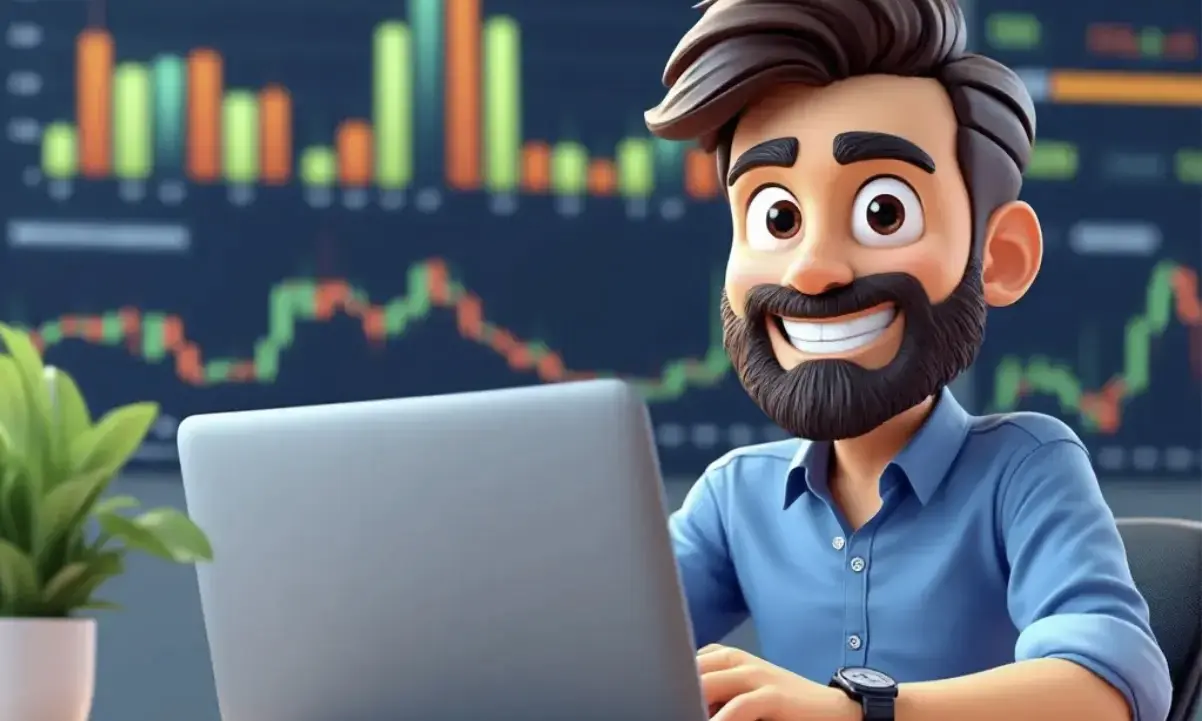
The OpenBB Platform is developed as open-source by the company OpenBB. In this article, we will highlight some features that we frequently use on the OpenBB Platform.
What is the OpenBB Platform?
The OpenBB Platform is a modular ecosystem. It enables access to around 100 data sources from different asset classes. In general, the platform consists of the OpenBB core and extensions. Some extensions are directly from the OpenBB team, and others are from the community.
The OpenBB core simplifies the development of custom applications with optimized data provider connections. The extensions provide categorized data access and advanced features. This architecture makes the OpenBB Platform flexible.
The OpenBB Platform offers two interfaces: the Python interface and the Web API interface. The Python interface gives Python developers a smooth development experience.
The Web API allows any frontend to use the available endpoints of the OpenBB Platform easily. It also allows you to integrate the OpenBB Platform into any programming language or framework.
Get Started with the OpenBB Platform
To get started with the OpenBB Platform, you need to do a few things.
Installation
We recommend that you create a virtual Python environment. To do this, open a terminal of your choice. We use conda to create a virtual environment.
You can create a conda environment as follows:
- Create a conda environment (env): conda
create -n openbb-platform python=3.11.9
-> Answer the question Proceed ([y]/n)? with y. - Activate the environment:
conda activate openbb-platform
Now, your virtual environment is ready to use if you see no errors.
No errors? Awesome! 🥳
Next, we can install OpenBB from PyPi with the following command:
pip install openbb
This command installs only the base providers and extensions of the OpenBB Platform, so we can pick what we need. But you can also install everything in one wash by adding the [all]
flag.
OpenBB Hub
It is highly recommended to create an OpenBB Hub account, so you can keep all your API keys in one place. You can do this on the OpenBB Hub login page.
When you log in, you will see the following landing page.
Select the OpenBB Platform, and then, on the sidebar, you will see the “Credentials” section. There, you can manage your API Keys of different data providers.
In this article, we use API keys from the following data providers:
- FMP
- yfinance
- sec
Next, it makes sense to create a PAT (Personal Access Token) that we can use in our Python code to log into our OpenBB Hub account. Click on “Personal Access Token” in the sidebar and create your PAT.
You can log in from your Python environment with the following Code:
from openbb import obb
obb.account.login(pat="<YOUR_TOKEN>")
The OpenBB Platform has a modular structure and consists of several menus. There are utility ones or routers that serve as an entry point for different asset classes. In addition, there are many extensions for the OpenBB Platform.
You can display the current environment as follows:
obb
Result:
OpenBB Platform v4.3.3
Utilities:
/account
/user
/system
/coverage
Routers:
/commodity
/crypto
/currency
/derivatives
/economy
/equity
/etf
/fixedincome
/index
/news
/regulators
Extensions:
...
OpenBB Platform Documentation
There is well-structured documentation to help you find the right commands for your specific needs. On the documentation website, you can find all the menus and commands in the reference section.
Another great way to explore the commands is through the Python interface. If you type obb.
, your IntelliSense should show you what is available.
If you have found a specific command, you can add a question mark at the end of the command to see its signature, the docstring, and all the details. Look at the following example:
obb.equity.price.historical?
Result:
Signature:
obb.equity.price.historical(
symbol: Annotated[Union[str, List[str]], OpenBBField(description='Symbol to get data for. Multiple comma separated items allowed for provider(s): fmp, polygon, tiingo, yfinance.')],
start_date: Annotated[Union[datetime.date, NoneType, str], OpenBBField(description='Start date of the data, in YYYY-MM-DD format.')] = None,
end_date: Annotated[Union[datetime.date, NoneType, str], OpenBBField(description='End date of the data, in YYYY-MM-DD format.')] = None,
provider: Annotated[Optional[Literal['fmp', 'intrinio', 'polygon', 'tiingo', 'yfinance']], OpenBBField(description='The provider to use, by default None. If None, the priority list configured in the settings is used. Default priority: fmp, intrinio, polygon, tiingo, yfinance.')] = None,
**kwargs,
) -> openbb_core.app.model.obbject.OBBject
Docstring:
Get historical price data for a given stock. This includes open, high, low, close, and volume.
Parameters
----------
symbol : Union[str, List[str]]
Symbol to get data for. Multiple comma separated items allowed for provider(s): fmp, polygon, tiingo, yfinance.
...
Returns
-------
OBBject
results : List[EquityHistorical]
Serializable results.
provider : Optional[Literal['fmp', 'intrinio', 'polygon', 'tiingo', 'yfinance']]
Provider name.
warnings : Optional[List[Warning_]]
List of warnings.
chart : Optional[Chart]
Chart object.
extra : Dict[str, Any]
Extra info.
...
You see a detailed overview of the command. In addition, we get an OBBject as a return. In the next section, you will learn more about this object.
What is the OBBject?
The OBBject is the abbreviation for OpenBB result object and is a standardized object. It contains the results, warnings, charts, and more.
OBBject
results : List[EquityHistorical]
Serializable results.
provider : Optional[Literal['fmp', 'intrinio', 'polygon', 'tiingo', 'yfinance']]
Provider name.
warnings : Optional[List[Warning_]]
List of warnings.
chart : Optional[Chart]
Chart object.
extra : Dict[str, Any]
Extra info.
One of the great things about OBBject is that you can present the results in different ways, such as to_df()
, to_json()
, and more.
Practical examples
The following examples show you how to use the OpenBB Platform for your investment research.
Working with stock market data
In our example, we use the OpenBB Platform to get the historical price data from Tesla. We can reach the command historical via the router equity and the subrouter price. In the Python code, it looks like this:
obb.equity.price.historical("TSLA")
Result:
OBBject
id: 067014b0-856b-71f6-8000-a6665a7b25d8
results: [{'date': datetime.date(2023, 10, 5), 'open': 260.0, 'high': 263.6, 'low':...
provider: fmp
warnings: None
chart: None
extra: {'metadata': {'arguments': {'provider_choices': {'provider': 'fmp'}, 'standa...
The result is an OBBject with all the information. Next, we can easily convert this data into a dataframe using the function to_df()
.
obb.equity.price.historical("TSLA").to_df().head()
Result:
We can also change the data provider. For example, we change it to ‘yfinance’.
obb.equity.price.historical("TSLA", provider="yfinance").to_df().head()
Result:
That is it. Next, you could process and plot the data.
Working with fundamental data
Another useful feature of the OpenBB Platform is retrieving fundamental data. In this example, we aim to obtain Tesla’s balance sheet. The process is similar to that used in the last section. Look at the following Python code:
obb.equity.fundamental.balance("TSLA")
Result:
OBBject
id: 067014c5-a8df-706f-8000-1953fc92e4eb
results: [{'period_ending': datetime.date(2023, 12, 31), 'fiscal_period': 'FY', 'fi...
provider: fmp
warnings: None
chart: None
extra: {'metadata': {'arguments': {'provider_choices': {'provider': 'fmp'}, 'standa...
We get an OBBject as a result. The default data provider is ‘fmp’ for the balance
command.
With the to_df()
function, we get the result as a dataframe again.
obb.equity.fundamental.balance("TSLA").to_df()
Result:
The result contains a lot of information. In the next steps, you could process this information according to your needs.
Analysis of two ETFs
In this section, we use the OpenBB Platform to analyze the iShares MSCI World ETF and the iShares MSCI ACWI ETF.
First, we must find out the symbols of the ETFs. To do this, you use the function obb.etf.search(...)
.
obb.etf.search(query="MSCI World ETF").to_df()
Result:
obb.etf.search(query="MSCI ACWI ETF").to_df()
Result:
Now, we have the symbols of the two ETFs. We can use these symbols to get the top holdings for each ETF.
MSCI World ETF
holdings = obb.etf.holdings("URTH", provider='sec').to_df()
holdings.head()
Result:
The top holdings of the MSCI World ETF are Microsoft, Apple, Nvidia, Amazon, and Meta. This ETF is very tech-heavy.
MSCI ACWI ETF
holdings = obb.etf.holdings("ACWI", provider='sec').to_df()
holdings.head()
Result:
The result for the MSCI ACWI ETF is very similar. The only difference is that Apple holds the largest position, while Microsoft holds the second largest position.
Next, we can analyze the price performance over different time periods. To do this, we use the function obb.etf.price_performance(...)
.
obb.etf.price_performance(symbol='URTH,ACWI', provider='fmp').to_df().transpose().head(10)
Result:
As a result, we see that the MSCI World ETF has outperformed the MSCI ACWI ETF over five years. The MSCI ACWI includes emerging markets as well as the industrialized countries from the MSCI World.
Conclusion
The OpenBB Platform is a modular ecosystem for accessing and analyzing financial data. In this article, we have shown you the basics of the OpenBB Platform.
To learn more about the OpenBB Platform, we recommend checking out the documentation and the notebook examples. Try out the examples to get familiar with how they work. As a data scientist or financial analyst, you will appreciate the OpenBB Platform.
💡 Do you enjoy our content and want to read super-detailed articles about data science topics? If so, be sure to check out our premium offer!